Several years ago I decided to make use of digital code signing certificates to sign the executable files of all my software products and also to sign the installers. As I’ve just had to renew my certificate I thought I’d write about it quickly here. Basically a digital code signing certificate allows you to fingerprint an executable file to assure you that a given file is from a given software publisher. Furthermore, it also checksums a file to prevent the executable file from being modified after it was signed.
Both of these are desirable from a user level because it helps to assure you that a file that is being downloaded is actually from who you expect it to be AND it hasn’t been modified to include something nefarious (such as virii or spyware). As a software publisher both of these things are useful because potential customers are more likely to actually install your software after they’ve downloaded it once it’s been signed correctly. It’s easy to tell if an executable file is digitally signed by the publisher. When you try to run the file after you’ve downloaded it from the internet Microsoft Windows will generally show you a message like this:
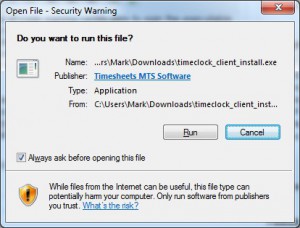
Windows Installation Warning Message
If the file is digitally signed then the name of the publisher will appear here. If you want to dig down deeper you can view the file properties of a signed file and you’ll see something like this:
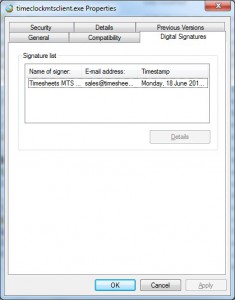
Signed File Properties
I use Comodo as the issuing body for the code signing certificates for my software. Rather than purchase directly from them I use one of their re-sellers, K-Software. The ordering process is painless and once you’ve placed an order Comodo will contact you for some identity documents to prove who you are. This is usually business registration documents but being in Australia I had to provide a bit more information and Comodo rang me as a further verification of my business identity. It should be comforting to the consumer that they go through this sort of process, as Comodo really does want to ensure that they only issue certificates to legitimate businesses.
One tip to anyone wanting to get one of these certificates is to not use Google Chrome or Mozilla Firefox when it comes time to actually download and install your code signing certificate. This is simply because the process within IE8 or better is fully automated and painless. Chrome and Firefox require you to jump through a few extra hoops to do the actual certificate installation and based on past experience it’s just not worth the hassle. Another tip is to ensure that you backup your certificate (which can be done via IE internet properties) somewhere safe so that you can move it to a new PC if required.
Actually signing your executable file and installer is pretty simple if you’re using Windows. I use the Microsoft command line tool signtool.exe that can be used to digitally sign files. This tool is part of the .NET SDK so you’ll need to ensure you’ve got that installed. Actually using signtool.exe is easy, there’s a line in all of my software build scripts that signs both the program executable file and software installer. The line looks something like this:
c:\code signing\signtool.exe" sign /a /t http://timestamp.comodoca.com/authenticode "C:\PathTo\Exe File\ProductName.exe